Nov
24
2015
By abernal
Arrays are collections of data.
Syntax
var passengers = ["Andres", "Paula","Carmenza","Jose"]; passengers[0]; // "Andres" passengers[2] = "Carmencita"; // ["Andres", "Paula","Carmencita","Jose"]
pop()
This function will take out the last element of the array, continue with our array "passengers" we can do the next :
var lastPassanger = passengers.pop(); alert("The last Passenger is :"+lastPassenger+" !"); // "The last Passenger is : Jose !" passengers; // ["Andres", "Paula","Carmencita"]
push()
This function will set the last element of the array, continue with our array "passengers" we can do the next :
passengers.push("Alejandro"); passengers; // ["Andres", "Paula","Carmencita", "Alejandro"]
map()
This function is useful in order to apply a certain operation for each of the elements of the array, returning an array with the result values.
Sample
var numbers = [1, 2, 3, 4, 5, 6]; var results = numbers.map(function (arrayCell) { return arrayCell * 2; } ); console.log(results); // This will print the array : [2, 4, 6, 8, 10, 12]
shift()
This method returns the firts value of the array (from which it was called) and then it will resize the length of the array
var fastPassQueue = ["Cedar Coaster", "Pines Plunge", "Birch Bumpers", "Pines Plunge"]; var firstItem = fastPassQueue.shift(); // this will take out the first element of the array, and it will return it to the variable console.log("This is the first Item of the array : "+firstItem);
Content
Arrays can contain a lot of stuff
- Strings
- Values
- Variables
- Other arrays
- A combination of the last ones
Multidimensional Arrays
The following image from (http://www.codeschools.com), ilustrates the idea
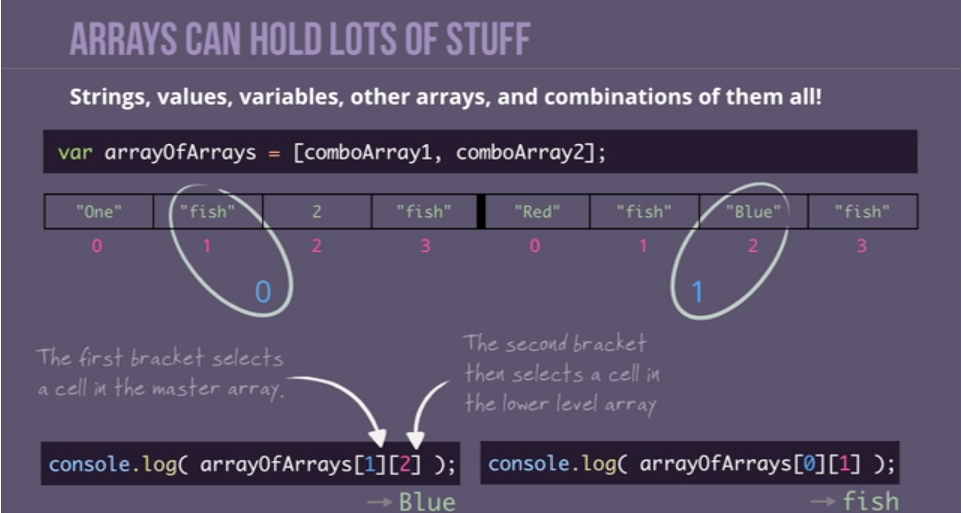
Remove an array Item
In order to remove an array Item we can follow the next procedure
var array = [1,2,3,4,5,6,7,8]; array[0] = undefined; array; // [undefined, 2, 3, 4,5,6,7,8]